Welcome to the world of React and Redux! This blog post is aimed at guiding you through the process of integrating Redux into a React application. Redux, a powerful state management tool, complements React's component-based architecture by providing a centralized store for your application's state. This setup leads to more predictable state management, especially in complex applications.
What is Redux?
Redux is an open-source JavaScript library for managing and centralizing application state. It's widely used with React but can be used with any other JavaScript framework or library. It's based on the Flux architecture and emphasizes a single, immutable state tree.
Why Use Redux with React?
- Predictable State Management: Redux enforces the use of pure functions, making the state predictable.
- Maintainability: It enhances maintainability by centralizing state management, making it easier to manage and debug.
- Developer Tools: Redux DevTools offer powerful tools for time-travel debugging and state observation.
- Community and Ecosystem: A strong community and a rich ecosystem of middleware and add-ons.
Setting Up Redux in a React App
To kick things off, let's set up a basic React application with Redux.
Installation
Create a new React app and install Redux and React-Redux.

Basic Example
-
Create a Redux Store:
First, let's create a simple Redux store. The store holds the complete state tree of your application.
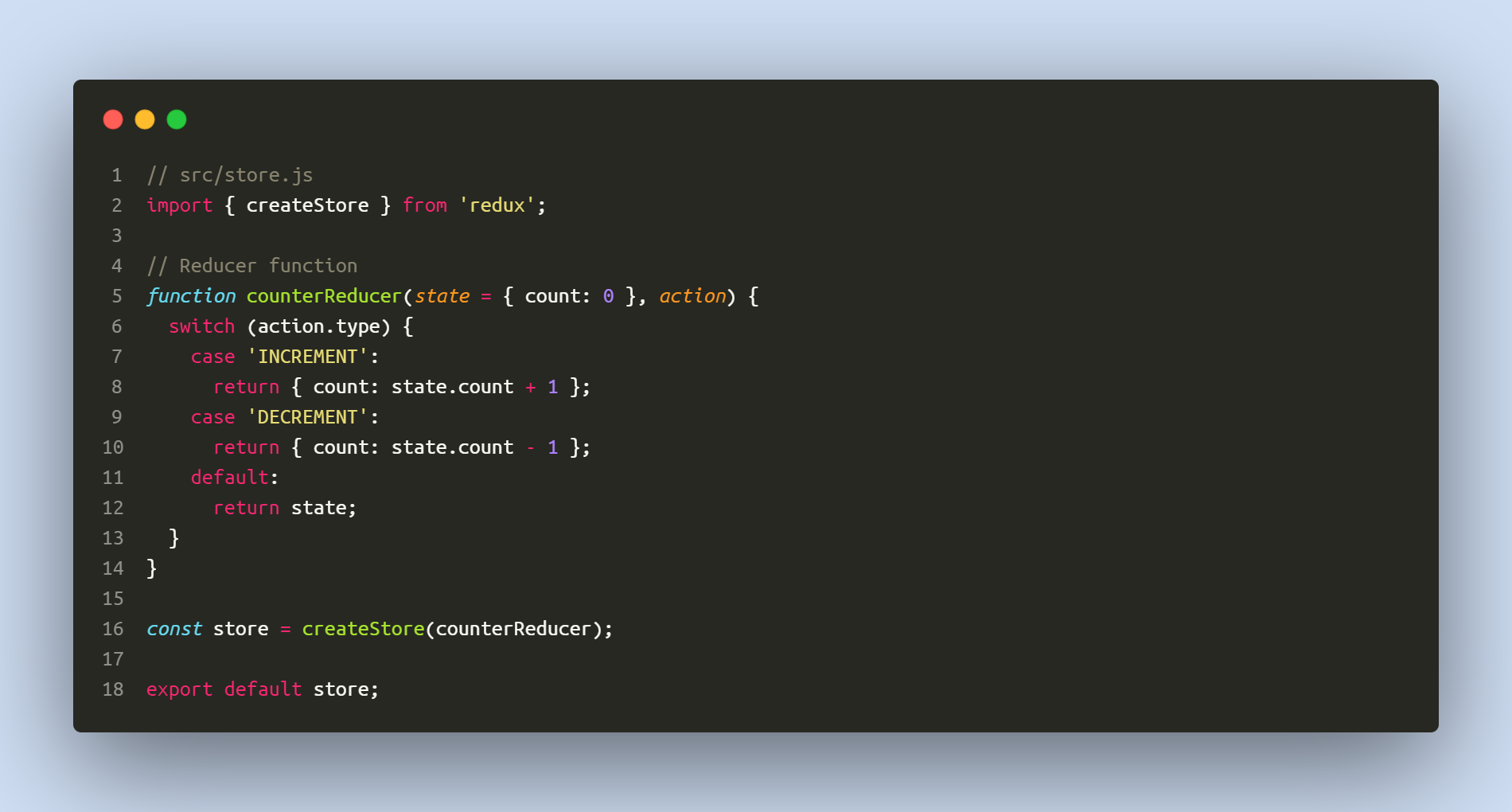
-
Integrating Redux with React:
Next, let's integrate Redux with our React application.
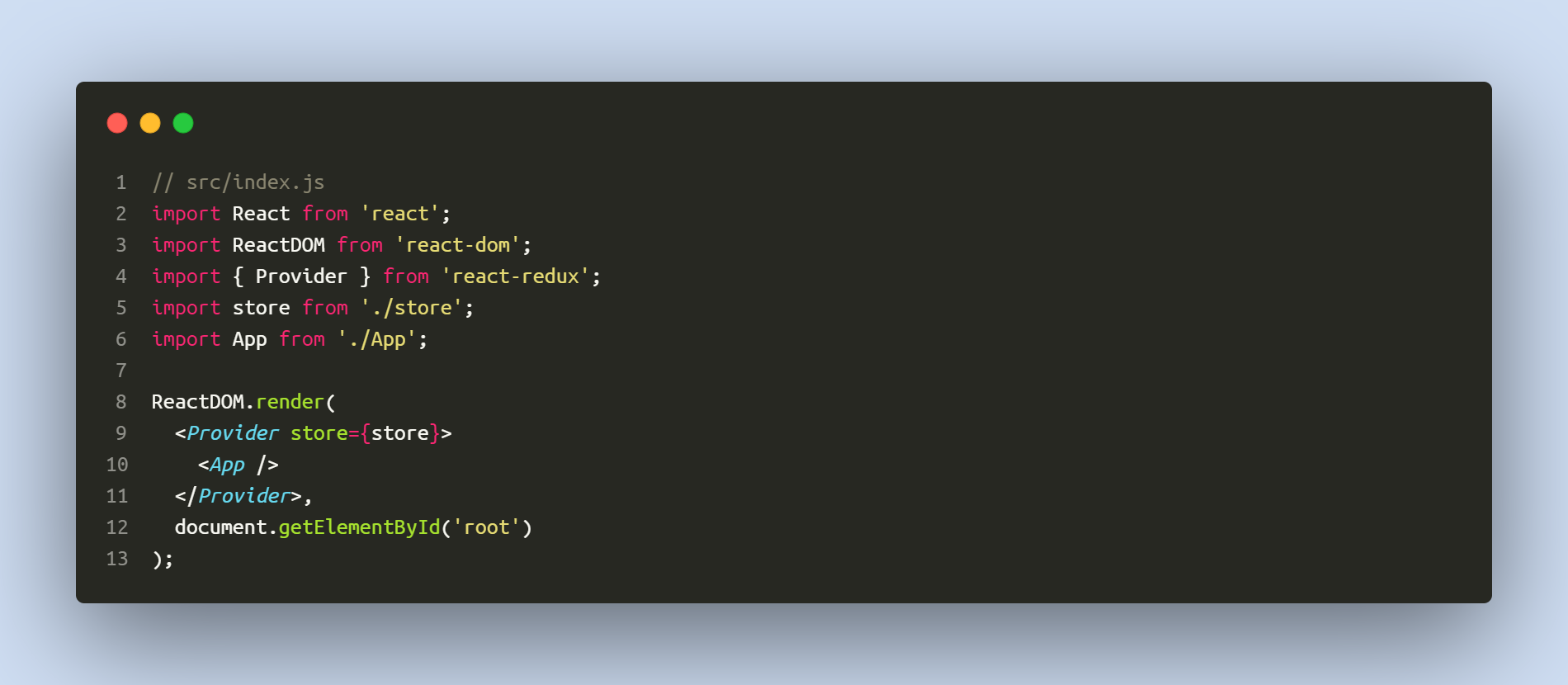
-
Connecting React Components to Redux:
Now, let's connect a React component to the Redux store.
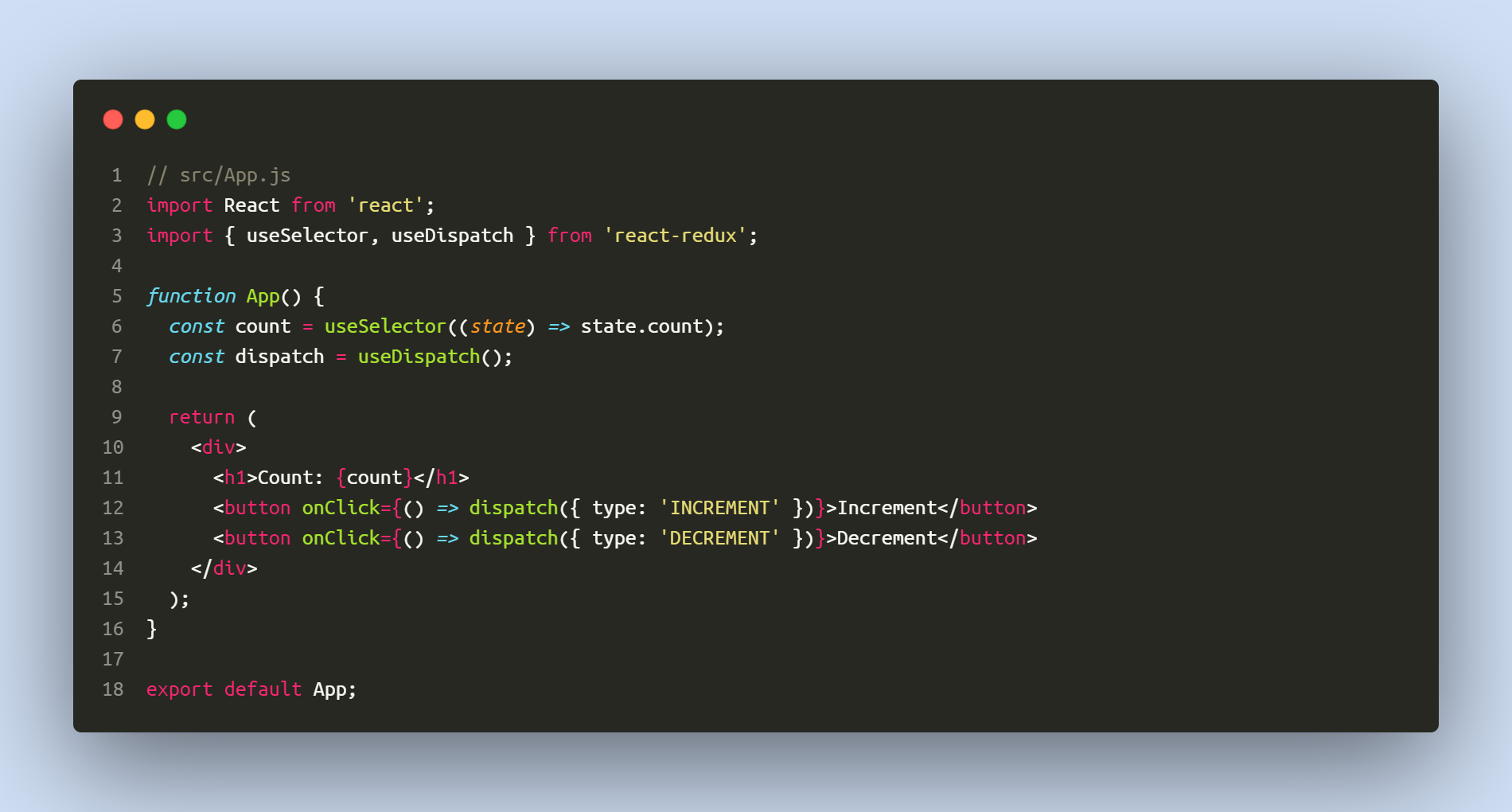
Understanding the Flow
- Action: A plain JavaScript object describing what happened. For example, { type: 'INCREMENT' }.
- Reducer: A function that determines how the state changes in response to an action.
- Store: The object that brings actions and reducers together. The store holds the application state.
Redux with Async code
What is Redux Thunk?
Redux Thunk is a middleware that lets you write action creators that return a function instead of an action. This is handy for handling side effects, such as asynchronous operations like API calls, in a Redux-based application.
Key Features of Redux Thunk:
- Handling Asynchronous Logic: Allows action creators to return functions instead of actions.
- Delaying Dispatch: Thunks can delay the dispatch of an action, or dispatch only if a certain condition is met.
- Access to Dispatch and State: The inner function receives the store's dispatch and getState methods as arguments.
Setting Up Redux Thunk
To use Redux Thunk, you need to install it and apply it as middleware to your Redux store.

Applying Middleware to Store
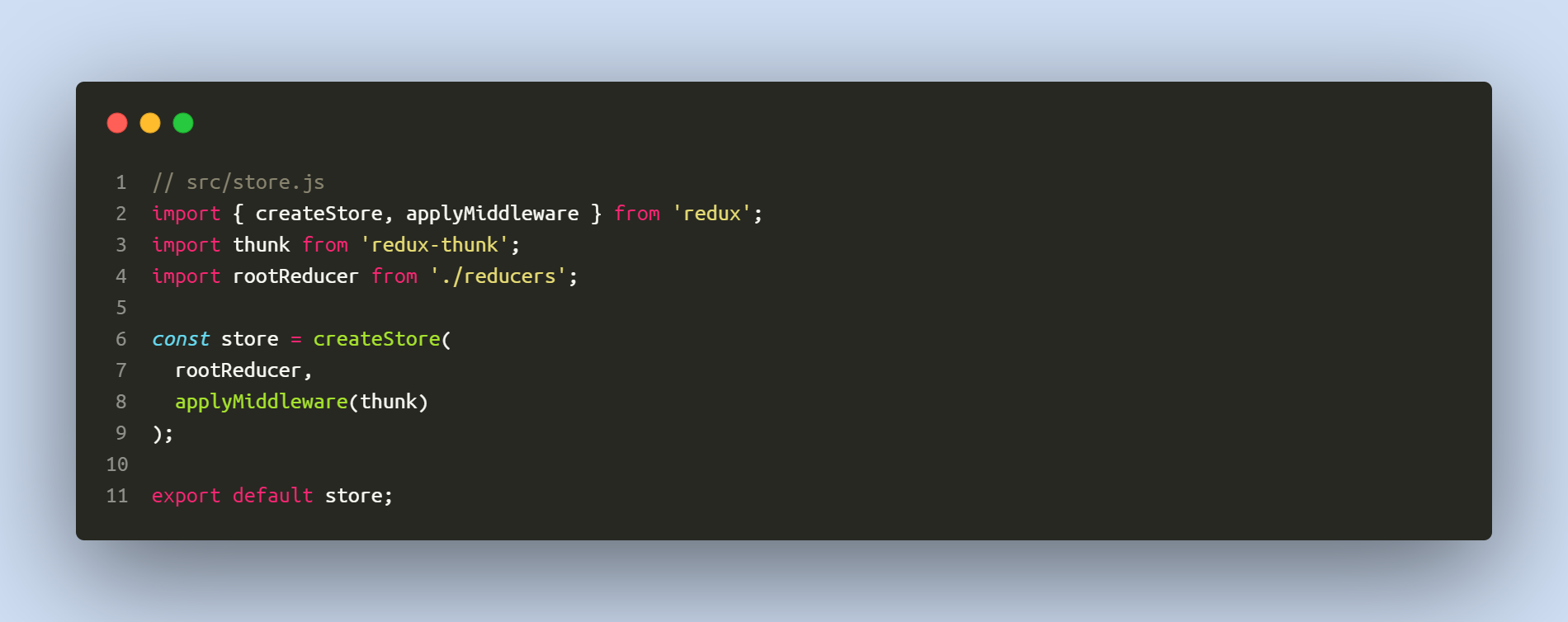
Using Redux Thunk for Asynchronous Actions
Let's create an asynchronous action creator using Redux Thunk to fetch data from an API.
Applying Middleware to Store
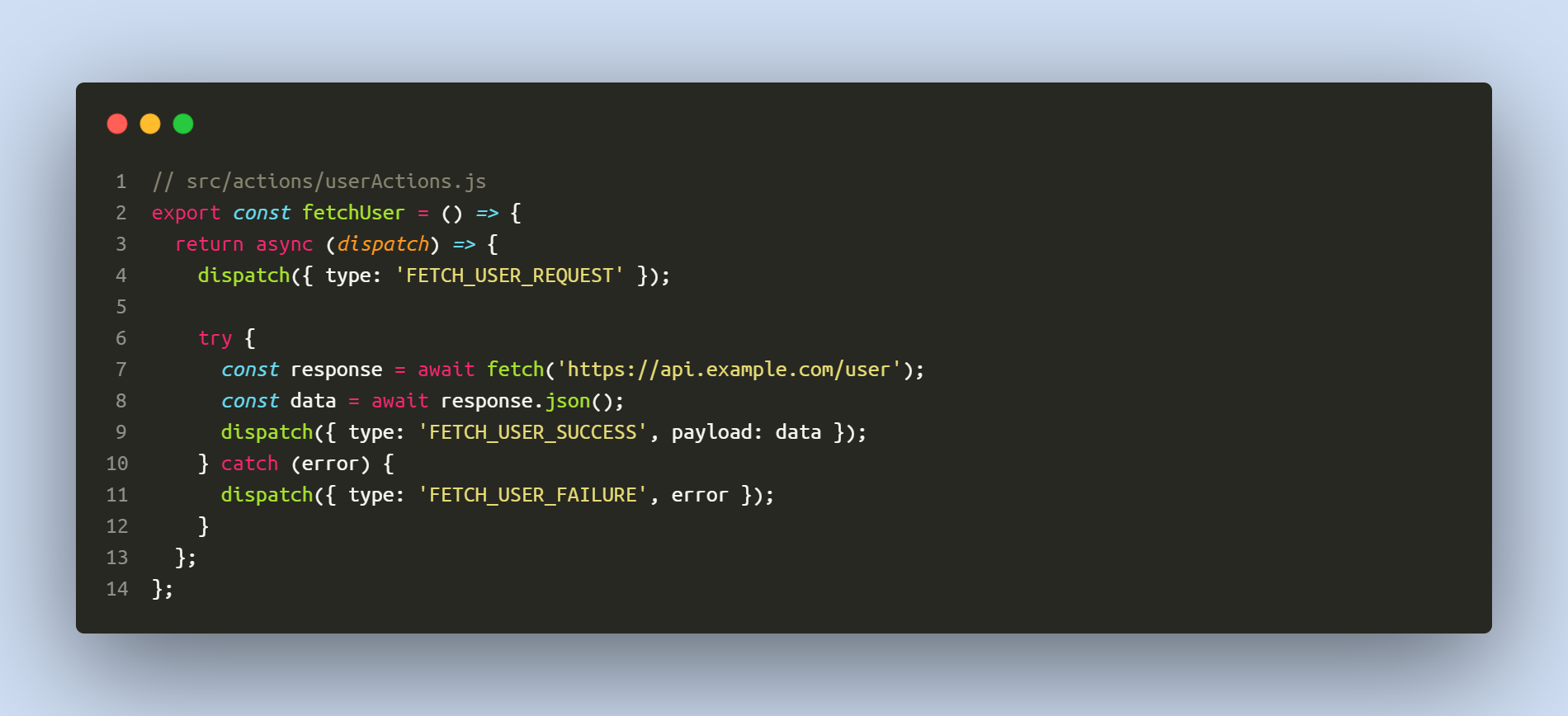
In this example, fetchUser
is an action creator that returns a thunk. This thunk performs the asynchronous API request and dispatches different actions based on the result of the API call.
Integrating Thunk Action into a React Component
To use this thunk action in a React component, you dispatch it using the useDispatch
hook.
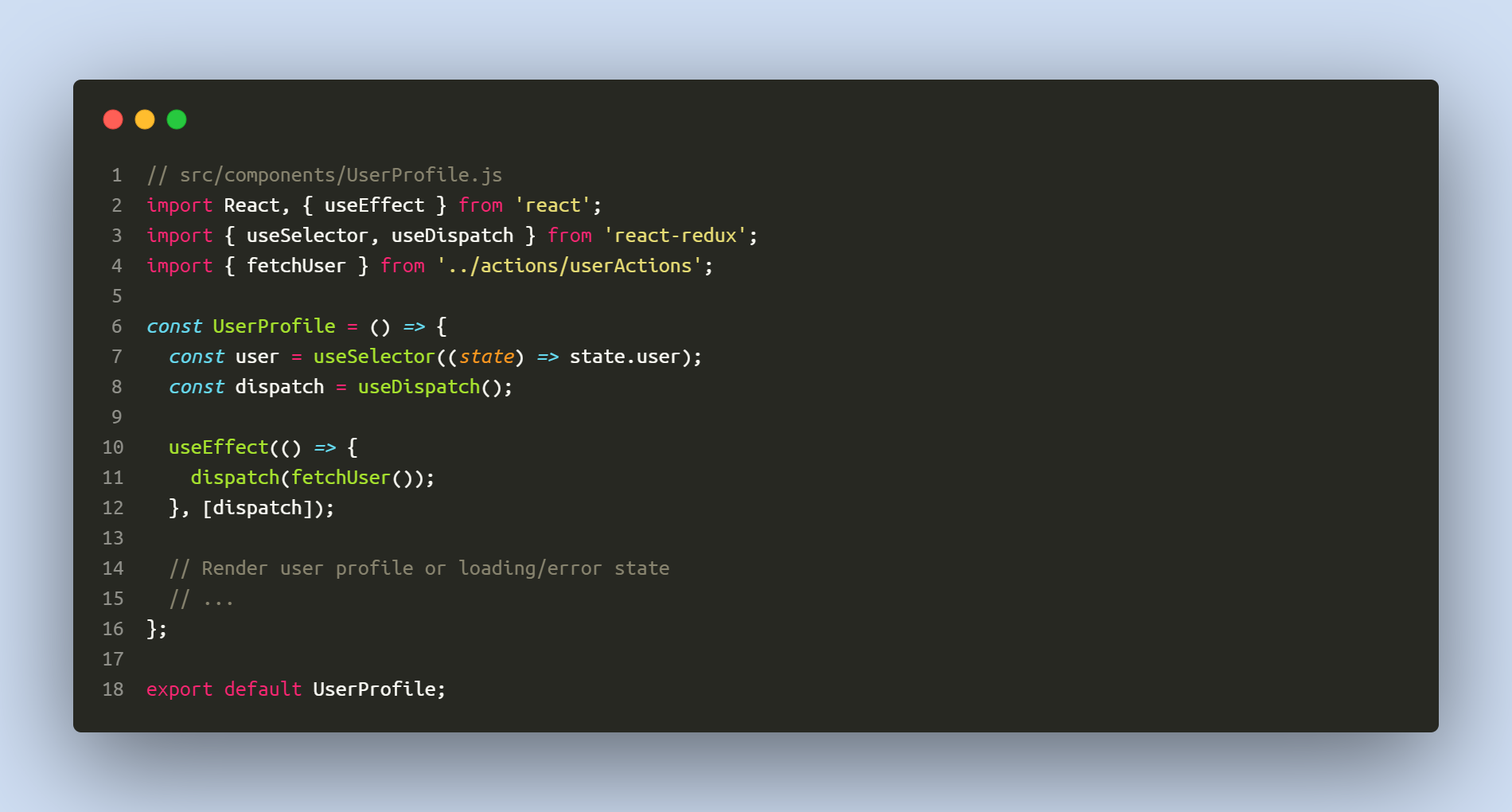
Advantages of Using Redux Thunk
- Simplicity: Redux Thunk is relatively straightforward to use and understand.
- Flexibility: It allows for more control over the dispatch process.
- Compatibility: Works well with other Redux ecosystem tools.
Embracing Redux Toolkit: My Preferred Way to Use Redux
Redux Toolkit (RTK) is the official, opinionated, batteries-included toolset for efficient Redux development. It's designed to simplify the process of writing Redux logic, addressing the common concerns of boilerplate and complexity. Let's delve into why Redux Toolkit is the preferred choice for many developers and how you can leverage it in your applications.
Why Choose Redux Toolkit?
- Simplifies Redux Code: Reduces boilerplate code significantly, making your Redux-related code cleaner and more manageable.
- Improves Readability: Offers a more straightforward approach to writing Redux logic, enhancing readability and maintainability.
- Built-In Best Practices: Comes with best practices built-in, ensuring high-quality Redux code.
- Powerful APIs: Provides APIs like
configureStore
, createReducer
, and createSlice
that abstract the complexities of setup and state management.
Setting Up Redux Toolkit
First, you need to add Redux Toolkit to your project.
npm install @reduxjs/toolkit react-redux
Core Concepts and Usage
Redux Toolkit simplifies many of the Redux tasks. Here are some of its core concepts:
-
Creating a Store with configureStore
configureStore
simplifies the store setup and automatically sets up the Redux DevTools extension and middleware like Redux Thunk.
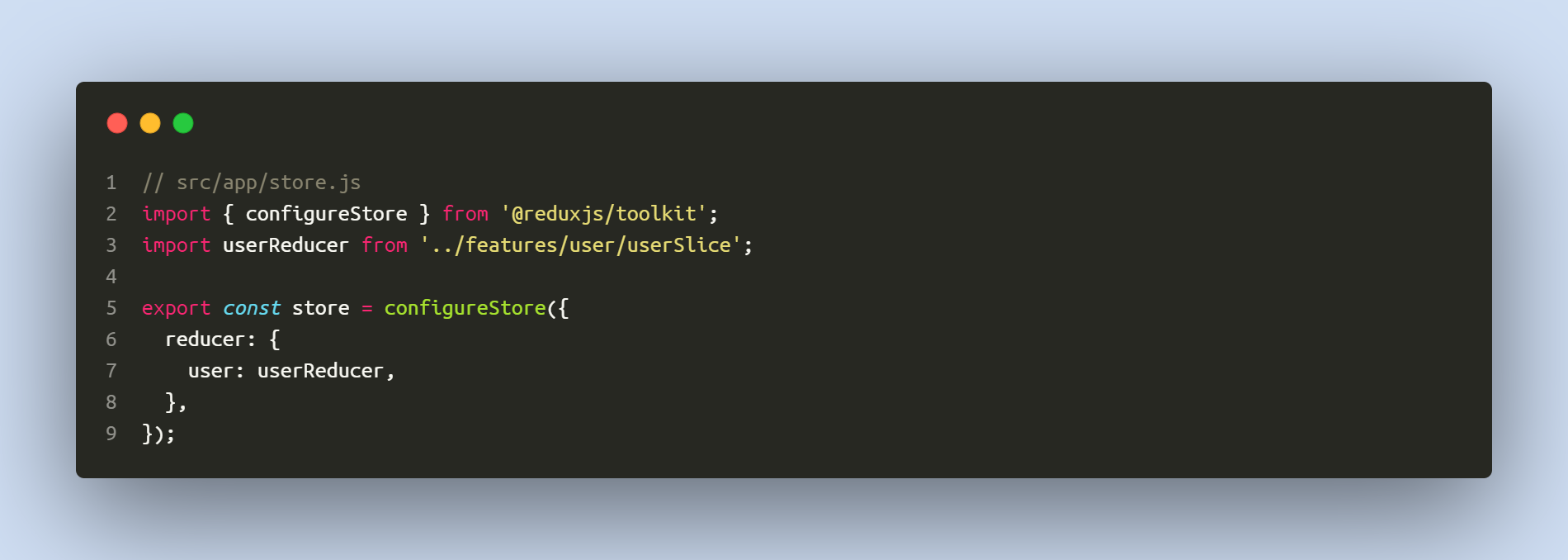
-
Defining State and Reducers with createSlice
createSlice
automatically generates action creators and action types corresponding to the reducers and state.
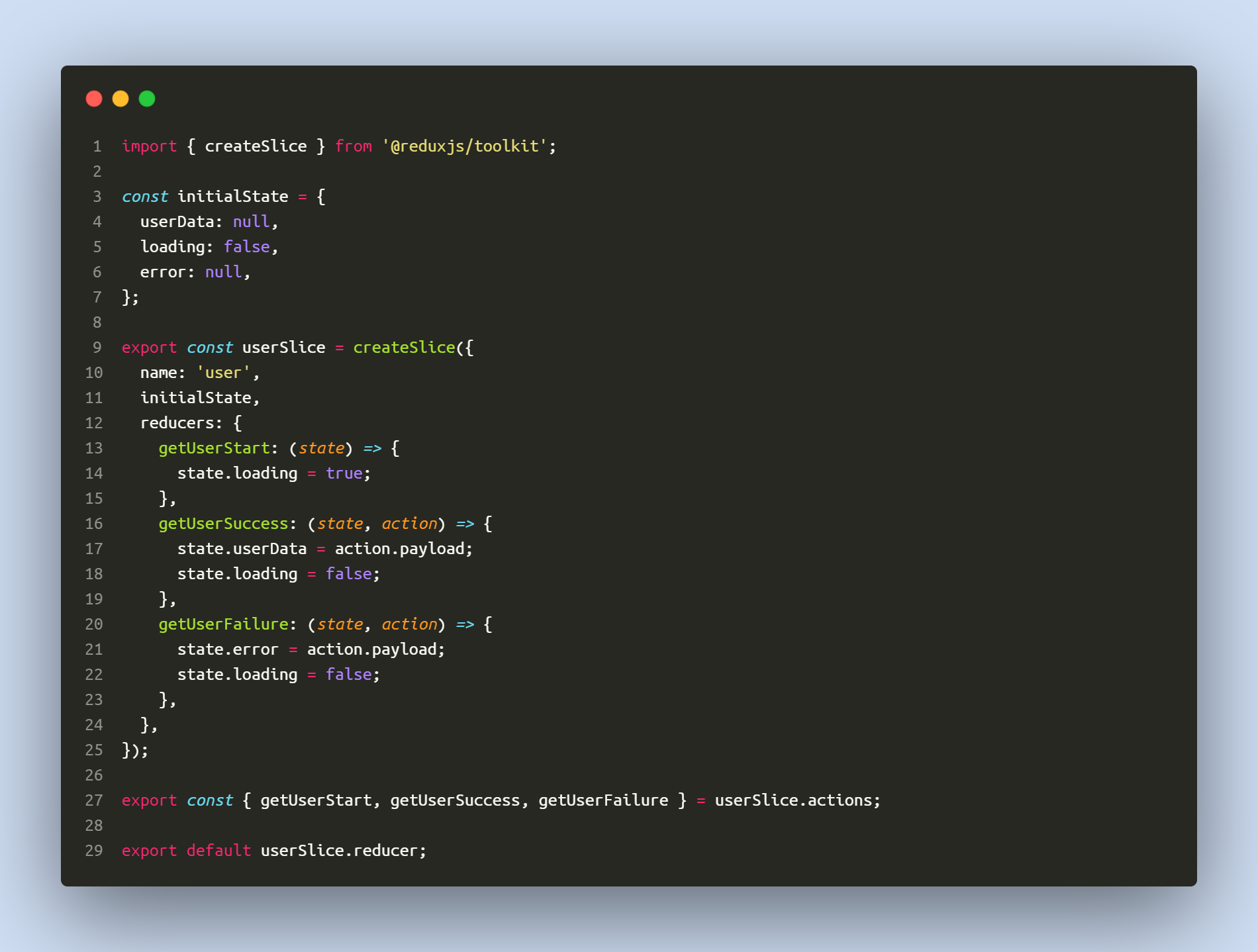
-
Async Thunks for Asynchronous Logic
Redux Toolkit simplifies async logic with createAsyncThunk
.
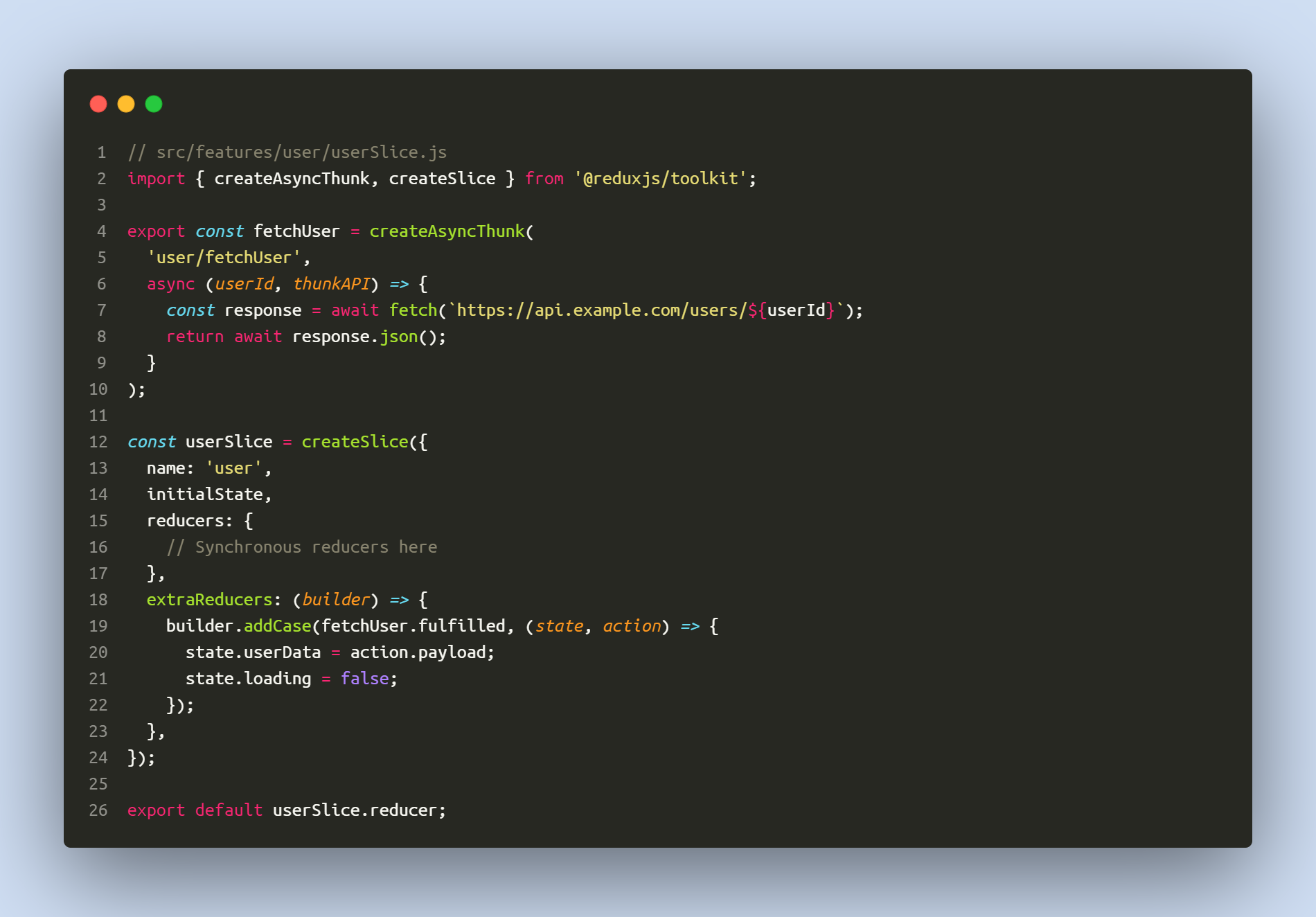
Benefits of Using Redux Toolkit
- Efficiency: Drastically reduces the amount of code needed to implement Redux.
- Consistency: Enforces best practices, ensuring consistency across your application.
- Productivity: Accelerates development, allowing you to focus more on business logic rather than boilerplate code.
- Modern Approach: Represents the modern, recommended approach to Redux, ensuring your application is up-to-date with the latest practices.
Conclusion
Redux Toolkit is a game-changer for Redux developers. It's not only a more efficient and productive way to use Redux but also ensures that your application adheres to the best practices in state management. With its powerful features and simplified API, Redux Toolkit is undoubtedly the preferred choice for modern Redux development.
Exploring Redux Toolkit Query (RTK Query) for Efficient Data Fetching
Redux Toolkit Query (RTK Query), part of the Redux Toolkit, is a powerful data fetching and caching tool. It simplifies the process of managing server state in your Redux applications. Let's delve into what makes RTK Query a go-to solution for efficient data fetching.
What is RTK Query?
RTK Query is a set of tools designed to manage data fetching, caching, synchronization, and updating of server state in applications. It's a highly productive, feature-rich library that abstracts away the complexities traditionally associated with data fetching and caching.
Key Features of RTK Query
- Automatic Caching: RTK Query automatically caches your data, reducing the need for redundant requests.
- Auto-Generated Hooks: It provides React hooks out of the box for data fetching operations.
- Polling and Real-Time Updates: Supports features like polling, query invalidation, and refetching, enabling real-time updates.
- Optimistic Updates: Facilitates optimistic updates for a smoother user experience.
- Minimal Boilerplate: Significantly reduces the boilerplate code required for data fetching and state management.
Setting Up RTK Query
To get started, you need to configure an API slice using createApi.
Installation
If you haven't already installed Redux Toolkit, do so along with RTK Query.

Creating an API Slice
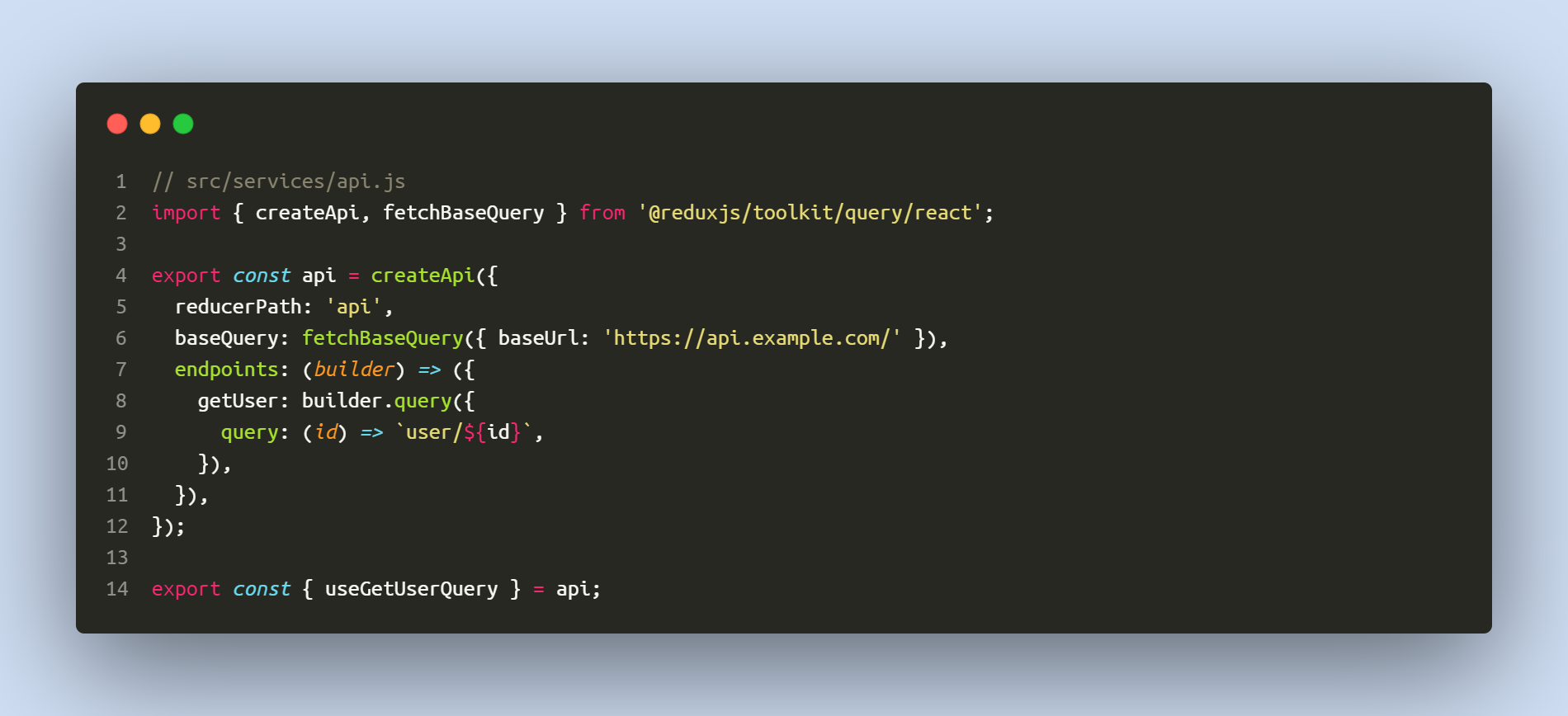
In this setup, we create an API slice with a getUser
endpoint for fetching user data.
Using RTK Query in a React Component
With the hooks auto-generated by RTK Query, you can easily fetch data in your components.
Example Usage in a Component
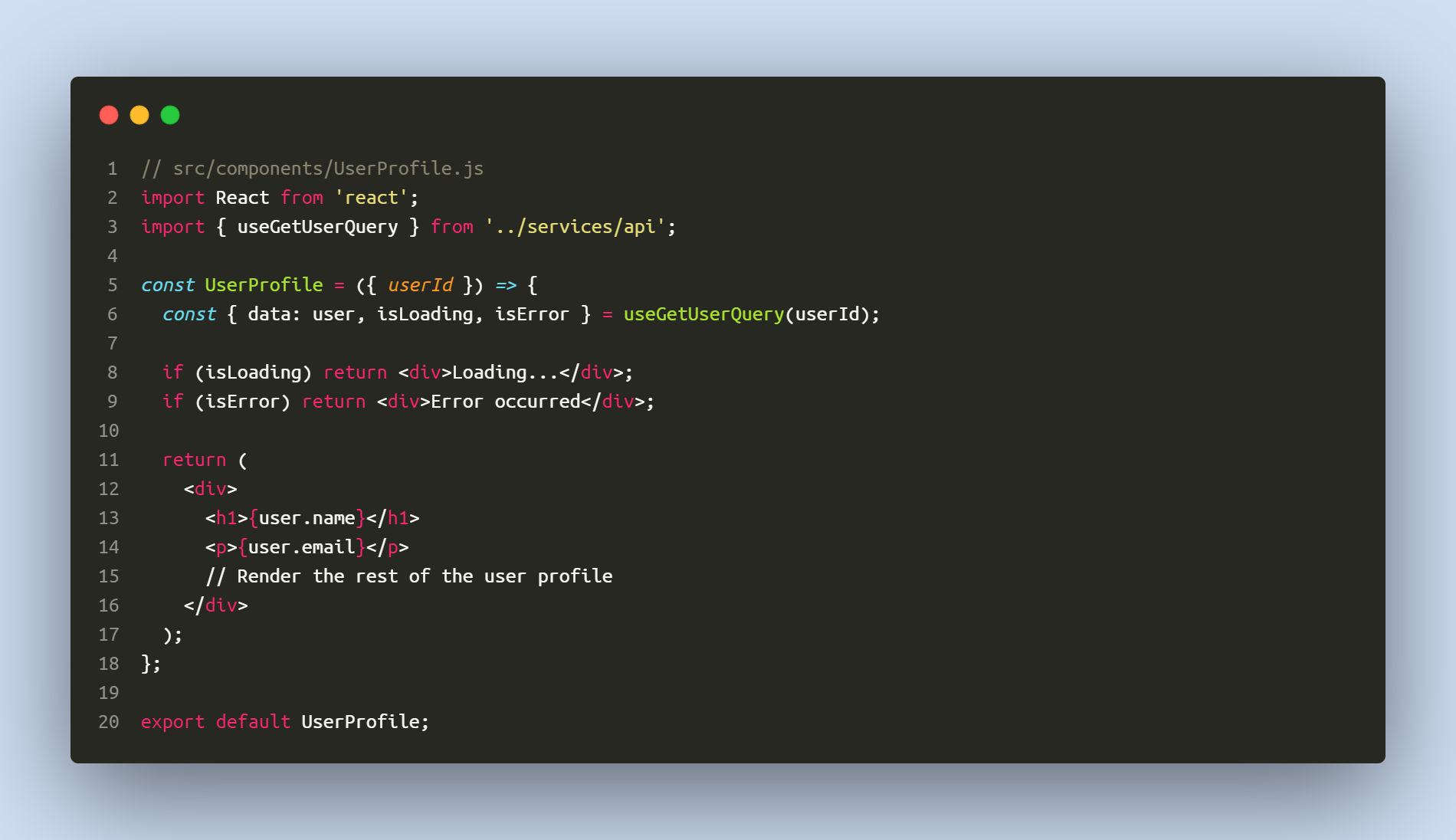
Benefits of Using RTK Query
- Simplified Data Management: RTK Query handles the complexities of loading, caching, refreshing, and updating server-side data.
- Performance Optimization: With its caching mechanism, RTK Query minimizes the number of server requests, improving performance.
- Developer Experience: Auto-generation of hooks and minimal boilerplate code make it a delight to use.
- Seamless Integration with Redux: It integrates neatly into the Redux ecosystem, complementing your existing state management.
RTK Query is a robust tool for efficiently managing server-side data in Redux applications. It streamlines the data fetching process, reducing the amount of code you need to write and maintain, while providing powerful features like caching and real-time updates.
Comparing Redux Solutions with SWR and React Query
Redux, particularly with enhancements like Redux Toolkit and RTK Query, is a powerful state management tool for React applications. However, in the realm of data fetching and caching, other libraries like SWR and React Query have gained popularity. Let's compare these approaches to understand their strengths and ideal use cases.
Redux with Redux Toolkit and RTK Query
Redux, especially when combined with Redux Toolkit and RTK Query, offers a comprehensive solution for state management and data fetching.
Strengths:
- Centralized State Management: Redux provides a single source of truth for application state, making state management predictable and transparent.
- Scalability: Excellent for complex applications with intricate state management needs.
- Ecosystem and Community: A well-established community and a rich ecosystem of tools and middleware.
- RTK Query: Simplifies data fetching, caching, and synchronization, reducing boilerplate associated with these operations.
Ideal Use Cases:
- Large-scale applications with complex state management requirements.
- Projects where centralized state management is a priority.
- Applications that benefit from Redux's predictable state management and dev tools.
SWR (Stale-While-Revalidate)
SWR is a React hooks library for data fetching, created by Vercel. It focuses on providing a simple and efficient way to fetch, cache, and update data.
Strengths:
- Real-Time Data: SWR's strategy of stale-while-revalidate ensures data is constantly refreshed and up-to-date.
- Lightweight and Minimalist: Easy to set up and use, with less configuration overhead compared to Redux.
- Built-in Data Caching and Revalidation: Efficient data caching and automatic revalidation offer optimized performance.
- Suspense and Concurrent Mode Support: Designed to work seamlessly with React's modern features.
Ideal Use Cases:
- Applications requiring frequent data updates and real-time features.
- Projects where state management needs are primarily around data fetching.
- Lightweight applications or microservices where adding Redux might be overkill.
React Query
React Query is another library for fetching, caching, and updating data in React applications. It's often compared with SWR for its similar functionalities.
Strengths:
- Powerful Query Management: Advanced handling of queries and mutations, including query retries, pagination, and optimistic updates.
- Built-In DevTools: Offers dedicated dev tools for monitoring and managing queries.
- Flexibility: Adaptable to various fetching strategies and backend structures.
- Automatic Data Synchronization: Automatically updates queries in the background, ensuring data consistency.
Ideal Use Cases:
- Applications with complex data fetching requirements, such as handling dependent queries.
- Scenarios requiring advanced features like pagination, infinite queries, and optimistic updates.
- Projects where the primary concern is efficient data fetching and caching rather than global state management.
Conclusion
- Redux with RTK Query is ideal for complex applications where robust state management is crucial, and data fetching is part of a larger state management picture.
- SWR shines in scenarios where real-time data and frequent updates are key, with a focus on simplicity and minimal configuration.
- React Query excels in applications with complex data fetching needs, requiring advanced features like query management and automatic synchronization.
The choice between these tools largely depends on your project's specific requirements and the aspects of state management and data fetching you prioritize. Each offers unique benefits, and understanding these can guide you to the right tool for your application's needs.